Introduction
This is the Egon API documentation.
This documentation aims to provide all the information you need to work with our API.
Base URL
https://api.egon.com
Authenticating requests
To authenticate requests, include an Authorization
header with the value "Bearer {YOUR_APP_KEY}"
.
All authenticated endpoints are marked with a requires authentication
badge in the documentation below.
You can view and manage your API keys in the Egon Apps.
Bearer authentication (also called token authentication) is an HTTP authentication scheme that involves security tokens called bearer tokens. The name “Bearer authentication” can be understood as “give access to the bearer of this token.” The bearer token is a cryptic string, usually generated by the server in response to a login request. The client must send this token in the Authorization header when making requests to protected resources.
The access token is passed with all further requests to the API using the Authorization
header, like so:
Authorization: Bearer {token}
Suggest V3
Address (full)
requires authentication
Suggestion for full address
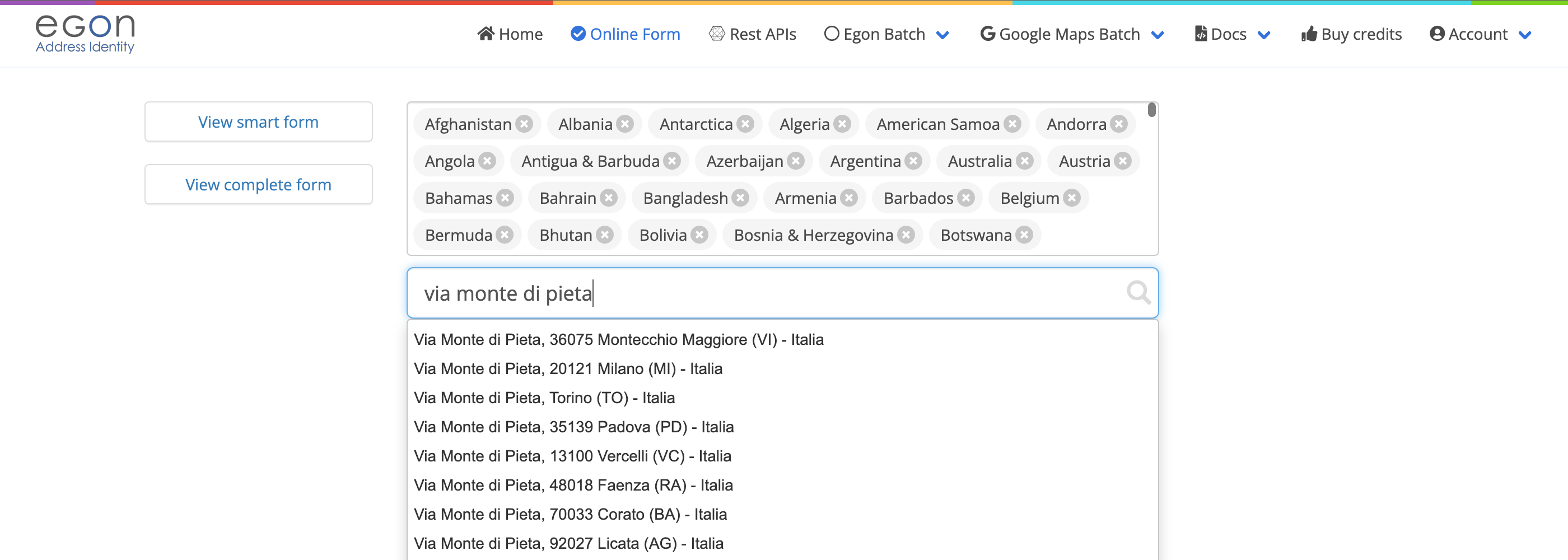
Example request:
curl --request GET \
--get "https://api.egon.com/v3/suggest/address?query=Via+Roveggia+5c&restrict_id=38000000001&restrict_level=country" \
--header "Authorization: Bearer {YOUR_APP_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$response = $client->get(
'https://api.egon.com/v3/suggest/address',
[
'headers' => [
'Authorization' => 'Bearer {YOUR_APP_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'query'=> 'Via Roveggia 5c',
'restrict_id'=> '38000000001',
'restrict_level'=> 'country',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://api.egon.com/v3/suggest/address"
);
const params = {
"query": "Via Roveggia 5c",
"restrict_id": "38000000001",
"restrict_level": "country",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"Authorization": "Bearer {YOUR_APP_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
[
{
"iso3": "ITA",
"level": "street",
"id": 38000073665,
"country": "Italia",
"region": "Veneto",
"province": "Verona",
"province_code": "VR",
"city": "Verona",
"zipcode": "37136",
"street": "Via Roveggia",
"number": "5",
"exponent": "c",
"label": "Via Roveggia 5c, 37136 Verona (VR) - Italia"
},
{
"iso3": "ITA",
"level": "street",
"id": 38000860896,
"country": "Italia",
"region": "Puglia",
"province": "Taranto",
"province_code": "TA",
"city": "Manduria",
"district1": "Torre Colimena",
"zipcode": "74024",
"street": "Via 5C",
"number": "5",
"exponent": "c",
"label": "Via 5C 5c, 74024 Manduria (TA) - Italia"
},
{
"iso3": "ECU",
"level": "street",
"id": 21800019148,
"country": "Ecuador",
"province": "Pichincha",
"city": "Cayambe",
"street": "5C",
"number": "5",
"exponent": "c",
"label": "5C 5c, Cayambe - Ecuador"
},
{
"iso3": "ECU",
"level": "street",
"id": 21800012971,
"country": "Ecuador",
"province": "Azuay",
"city": "Cuenca",
"street": "5C",
"number": "5",
"exponent": "c",
"label": "5C 5c, Cuenca - Ecuador"
},
{
"iso3": "ECU",
"level": "street",
"id": 21800019281,
"country": "Ecuador",
"province": "Pichincha",
"city": "Quito",
"street": "5C",
"number": "5",
"exponent": "c",
"label": "5C 5c, Quito - Ecuador"
},
{
"iso3": "MEX",
"level": "street",
"id": 48404240605,
"country": "México",
"state": "Yucatán",
"state_code": "YUC",
"city": "Kanasín",
"zipcode": "97370",
"street": "Calle 5C",
"number": "5",
"exponent": "c",
"label": "Calle 5C 5c, 97370 Kanasín - México"
},
{
"iso3": "MEX",
"level": "street",
"id": 48404245442,
"country": "México",
"state": "Yucatán",
"state_code": "YUC",
"city": "Mérida",
"zipcode": "97130",
"street": "Calle 5C",
"number": "5",
"exponent": "c",
"label": "Calle 5C 5c, 97130 Mérida - México"
},
{
"iso3": "MEX",
"level": "street",
"id": 48404245443,
"country": "México",
"state": "Yucatán",
"state_code": "YUC",
"city": "Mérida",
"zipcode": "97133",
"street": "Calle 5C",
"number": "5",
"exponent": "c",
"label": "Calle 5C 5c, 97133 Mérida - México"
},
{
"iso3": "MEX",
"level": "street",
"id": 48404245444,
"country": "México",
"state": "Yucatán",
"state_code": "YUC",
"city": "Mérida",
"zipcode": "97204",
"street": "Calle 5C",
"number": "5",
"exponent": "c",
"label": "Calle 5C 5c, 97204 Mérida - México"
},
{
"iso3": "MEX",
"level": "street",
"id": 48404245445,
"country": "México",
"state": "Yucatán",
"state_code": "YUC",
"city": "Mérida",
"zipcode": "97217",
"street": "Calle 5C",
"number": "5",
"exponent": "c",
"label": "Calle 5C 5c, 97217 Mérida - México"
}
]
Example response (401, Unauthorized):
{
"error": {
"code": 401,
"message": "You did not provide an API key. You need to provide your API key in the Authorization header, using Bearer auth (e.g. 'Authorization: Bearer {token}'). See https://account.egon.com/docs for details."
}
}
Example response (400, Bad Request):
{
"error": {
"code": 400,
"message": "Missing the 'query'."
}
}
Received response:
Request failed with error:
Response
Response Fields
iso3
string
Country code (ISO 3166-1 alpha-3).
level
string
Territorial level of the element.
id
integer
Element ID.
country
string
Country name.
state
string
(optional) State.
state_code
string
(optional) State code.
region
string
(optional) Region.
region_code
string
(optional) Region code.
province
string
(optional) Province.
province_code
string
(optional) Province code.
city
string
City.
district1
string
(optional) Sub-locality District level 1.
zipcode
string
(optional) Postal code.
street
string
Street name.
number
string
House number.
exponent
string
Exponent.
label
string
Complete address on single line.
It contains the main territorial levels that can be showed as a label.
Country
requires authentication
Suggestion for country
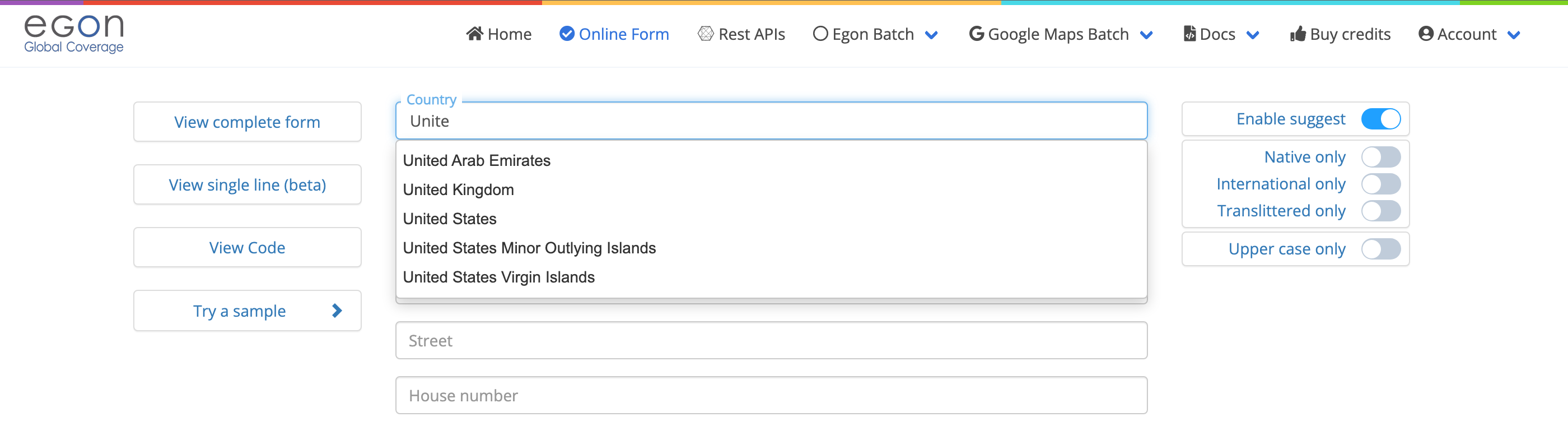
Example request:
curl --request GET \
--get "https://api.egon.com/v3/suggest/country?query=Italia" \
--header "Authorization: Bearer {YOUR_APP_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$response = $client->get(
'https://api.egon.com/v3/suggest/country',
[
'headers' => [
'Authorization' => 'Bearer {YOUR_APP_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'query'=> 'Italia',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://api.egon.com/v3/suggest/country"
);
const params = {
"query": "Italia",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"Authorization": "Bearer {YOUR_APP_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
[
{
"iso3": "ITA",
"level": "country",
"id": 38000000001,
"country": "Italia"
}
]
Example response (401, Unauthorized):
{
"error": {
"code": 401,
"message": "You did not provide an API key. You need to provide your API key in the Authorization header, using Bearer auth (e.g. 'Authorization: Bearer {token}'). See https://account.egon.com/docs for details."
}
}
Received response:
Request failed with error:
Response
Response Fields
level
string
Territorial level of the element.
id
integer
Element ID.
iso3
string
Country code (ISO 3166-1 alpha-3).
country
string
Country name.
State
requires authentication
Suggestion for state
Example request:
curl --request GET \
--get "https://api.egon.com/v3/suggest/state?query=California&iso3=USA" \
--header "Authorization: Bearer {YOUR_APP_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$response = $client->get(
'https://api.egon.com/v3/suggest/state',
[
'headers' => [
'Authorization' => 'Bearer {YOUR_APP_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'query'=> 'California',
'iso3'=> 'USA',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://api.egon.com/v3/suggest/state"
);
const params = {
"query": "California",
"iso3": "USA",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"Authorization": "Bearer {YOUR_APP_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
[
{
"iso3": "USA",
"level": "state",
"id": 84000000010,
"country": "United States",
"state": "California",
"state_code": "CA"
}
]
Example response (401, Unauthorized):
{
"error": {
"code": 401,
"message": "You did not provide an API key. You need to provide your API key in the Authorization header, using Bearer auth (e.g. 'Authorization: Bearer {token}'). See https://account.egon.com/docs for details."
}
}
Received response:
Request failed with error:
Response
Response Fields
level
string
Territorial level of the element.
id
integer
Element ID.
iso3
string
Country code (ISO 3166-1 alpha-3).
country
string
Country name.
state
string
(optional) State.
state_code
string
(optional) State code.
zipcode
string
(optional) Postal code.
Region
requires authentication
Suggestion for region
Example request:
curl --request GET \
--get "https://api.egon.com/v3/suggest/region?query=Veneto&iso3=ITA" \
--header "Authorization: Bearer {YOUR_APP_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$response = $client->get(
'https://api.egon.com/v3/suggest/region',
[
'headers' => [
'Authorization' => 'Bearer {YOUR_APP_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'query'=> 'Veneto',
'iso3'=> 'ITA',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://api.egon.com/v3/suggest/region"
);
const params = {
"query": "Veneto",
"iso3": "ITA",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"Authorization": "Bearer {YOUR_APP_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
[
{
"iso3": "ITA",
"level": "region",
"id": 38000000006,
"country": "Italia",
"region": "Veneto"
}
]
Example response (401, Unauthorized):
{
"error": {
"code": 401,
"message": "You did not provide an API key. You need to provide your API key in the Authorization header, using Bearer auth (e.g. 'Authorization: Bearer {token}'). See https://account.egon.com/docs for details."
}
}
Received response:
Request failed with error:
Response
Response Fields
level
string
Territorial level of the element.
id
integer
Element ID.
iso3
string
Country code (ISO 3166-1 alpha-3).
country
string
Country name.
state
string
(optional) State.
state_code
string
(optional) State code.
region
string
Region.
region_code
string
(optional) Region code.
zipcode
string
(optional) Postal code.
Province
requires authentication
Suggestion for province
Example request:
curl --request GET \
--get "https://api.egon.com/v3/suggest/province?query=Verbano&iso3=ITA&restrict_id=38000000001" \
--header "Authorization: Bearer {YOUR_APP_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$response = $client->get(
'https://api.egon.com/v3/suggest/province',
[
'headers' => [
'Authorization' => 'Bearer {YOUR_APP_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'query'=> 'Verbano',
'iso3'=> 'ITA',
'restrict_id'=> '38000000001',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://api.egon.com/v3/suggest/province"
);
const params = {
"query": "Verbano",
"iso3": "ITA",
"restrict_id": "38000000001",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"Authorization": "Bearer {YOUR_APP_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
[
{
"iso3": "ITA",
"level": "province",
"id": 38000000123,
"country": "Italia",
"region": "Piemonte",
"province": "Verbano Cusio Ossola",
"province_code": "VB"
}
]
Example response (401, Unauthorized):
{
"error": {
"code": 401,
"message": "You did not provide an API key. You need to provide your API key in the Authorization header, using Bearer auth (e.g. 'Authorization: Bearer {token}'). See https://account.egon.com/docs for details."
}
}
Received response:
Request failed with error:
Response
Response Fields
level
string
Territorial level of the element.
id
integer
Element ID.
iso3
string
Country code (ISO 3166-1 alpha-3).
country
string
Country name.
state
string
(optional) State.
state_code
string
(optional) State code.
region
string
(optional) Region.
region_code
string
(optional) Region code.
province
string
Province.
province_code
string
(optional) Province code.
zipcode
string
(optional) Postal code.
City
requires authentication
Suggestion for city
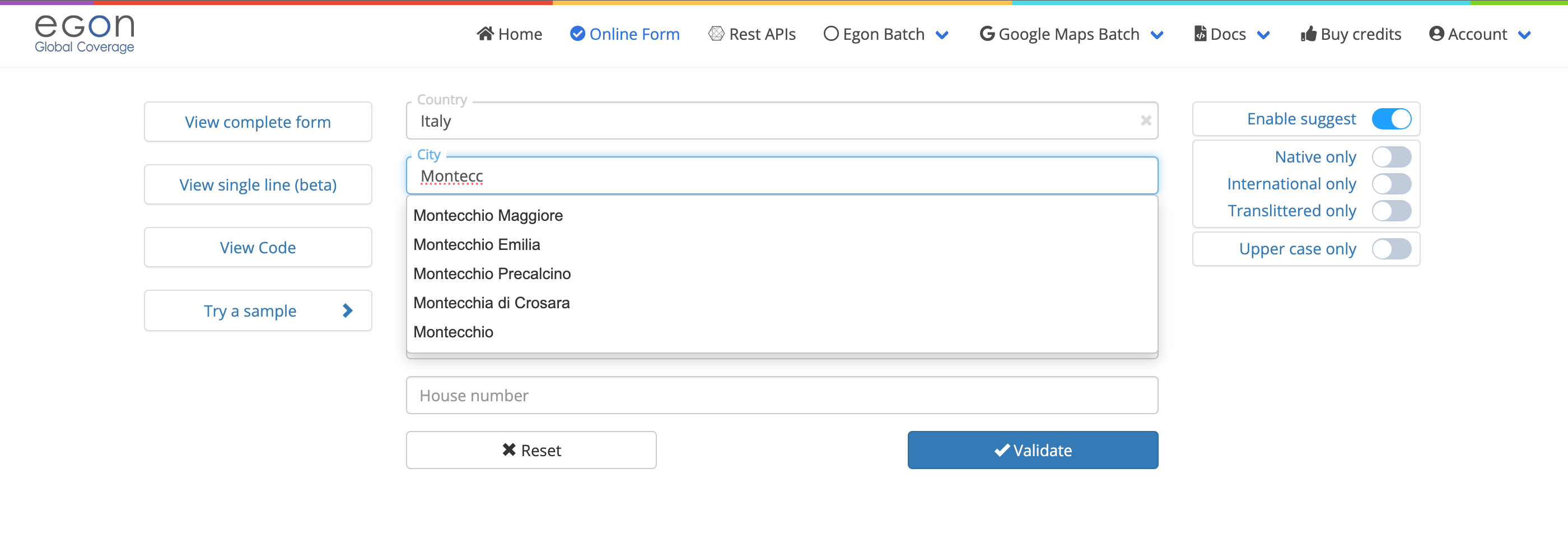
Example request:
curl --request GET \
--get "https://api.egon.com/v3/suggest/city?query=Vercelli&iso3=ITA&restrict_id=38000000001" \
--header "Authorization: Bearer {YOUR_APP_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$response = $client->get(
'https://api.egon.com/v3/suggest/city',
[
'headers' => [
'Authorization' => 'Bearer {YOUR_APP_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'query'=> 'Vercelli',
'iso3'=> 'ITA',
'restrict_id'=> '38000000001',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://api.egon.com/v3/suggest/city"
);
const params = {
"query": "Vercelli",
"iso3": "ITA",
"restrict_id": "38000000001",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"Authorization": "Bearer {YOUR_APP_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
[
{
"iso3": "ITA",
"level": "city",
"id": 38000001682,
"country": "Italia",
"region": "Piemonte",
"province": "Vercelli",
"province_code": "VC",
"city": "Vercelli",
"zipcode": "13100"
},
{
"iso3": "ITA",
"level": "city",
"id": 38000001606,
"country": "Italia",
"region": "Piemonte",
"province": "Vercelli",
"province_code": "VC",
"city": "Borgo Vercelli",
"zipcode": "13012"
}
]
Example response (401, Unauthorized):
{
"error": {
"code": 401,
"message": "You did not provide an API key. You need to provide your API key in the Authorization header, using Bearer auth (e.g. 'Authorization: Bearer {token}'). See https://account.egon.com/docs for details."
}
}
Received response:
Request failed with error:
Response
Response Fields
level
string
Territorial level of the element.
id
integer
Element ID.
iso3
string
Country code (ISO 3166-1 alpha-3).
country
string
Country name.
state
string
(optional) State.
state_code
string
(optional) State code.
region
string
(optional) Region.
region_code
string
(optional) Region code.
province
string
(optional) Province.
province_code
string
(optional) Province code.
city
string
City.
zipcode
string
(optional) Postal code.
District1
requires authentication
Suggestion for district level 1
Example request:
curl --request GET \
--get "https://api.egon.com/v3/suggest/district1?query=Milano&iso3=ITA&restrict_id=38000000001" \
--header "Authorization: Bearer {YOUR_APP_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$response = $client->get(
'https://api.egon.com/v3/suggest/district1',
[
'headers' => [
'Authorization' => 'Bearer {YOUR_APP_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'query'=> 'Milano',
'iso3'=> 'ITA',
'restrict_id'=> '38000000001',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://api.egon.com/v3/suggest/district1"
);
const params = {
"query": "Milano",
"iso3": "ITA",
"restrict_id": "38000000001",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"Authorization": "Bearer {YOUR_APP_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
[
{
"iso3": "ITA",
"level": "district1",
"id": 38001363482,
"country": "Italia",
"region": "Umbria",
"province": "Perugia",
"province_code": "PG",
"city": "Spoleto",
"district1": "Milano",
"zipcode": "06049"
},
{
"iso3": "ITA",
"level": "district1",
"id": 38000022330,
"country": "Italia",
"region": "Lombardia",
"province": "Milano",
"province_code": "MI",
"city": "Segrate",
"district1": "Milano Due",
"zipcode": "20054"
},
{
"iso3": "ITA",
"level": "district1",
"id": 38000009426,
"country": "Italia",
"region": "Emilia-Romagna",
"province": "Ravenna",
"province_code": "RA",
"city": "Cervia",
"district1": "Milano Marittima",
"zipcode": "48015"
},
{
"iso3": "ITA",
"level": "district1",
"id": 38000019401,
"country": "Italia",
"region": "Lombardia",
"province": "Milano",
"province_code": "MI",
"city": "Basiglio",
"district1": "Milano Tre",
"zipcode": "20079"
},
{
"iso3": "ITA",
"level": "district1",
"id": 38001129710,
"country": "Italia",
"region": "Lombardia",
"province": "Milano",
"province_code": "MI",
"city": "Assago",
"district1": "Milanofiori",
"zipcode": "20057"
},
{
"iso3": "ITA",
"level": "district1",
"id": 38001308925,
"country": "Italia",
"region": "Piemonte",
"province": "Torino",
"province_code": "TO",
"city": "Torino",
"district1": "Barriera di Milano",
"zipcode": "10100"
},
{
"iso3": "ITA",
"level": "district1",
"id": 38001131341,
"country": "Italia",
"region": "Veneto",
"province": "Verona",
"province_code": "VR",
"city": "Verona",
"district1": "Borgo Milano",
"zipcode": "37100"
},
{
"iso3": "ITA",
"level": "district1",
"id": 38001129711,
"country": "Italia",
"region": "Lombardia",
"province": "Milano",
"province_code": "MI",
"city": "Assago",
"district1": "Centro Congressi Milanofiori",
"zipcode": "20057"
},
{
"iso3": "ITA",
"level": "district1",
"id": 38000017703,
"country": "Italia",
"region": "Trentino-Alto Adige",
"province": "Trento",
"province_code": "TN",
"city": "Sporminore",
"district1": "Maso Milano",
"zipcode": "38010"
},
{
"iso3": "ITA",
"level": "district1",
"id": 38000022199,
"country": "Italia",
"region": "Lombardia",
"province": "Lodi",
"province_code": "LO",
"city": "Tavazzano con Villavesco",
"district1": "Muzza di Milano",
"zipcode": "26838"
}
]
Example response (401, Unauthorized):
{
"error": {
"code": 401,
"message": "You did not provide an API key. You need to provide your API key in the Authorization header, using Bearer auth (e.g. 'Authorization: Bearer {token}'). See https://account.egon.com/docs for details."
}
}
Received response:
Request failed with error:
Response
Response Fields
level
string
Territorial level of the element.
id
integer
Element ID.
iso3
string
Country code (ISO 3166-1 alpha-3).
country
string
Country name.
state
string
(optional) State.
state_code
string
(optional) State code.
region
string
(optional) Region.
region_code
string
(optional) Region code.
province
string
(optional) Province.
province_code
string
(optional) Province code.
city
string
City.
district1
string
Sub-locality District level 1.
zipcode
string
(optional) Postal code.
Street
requires authentication
Suggestion for street
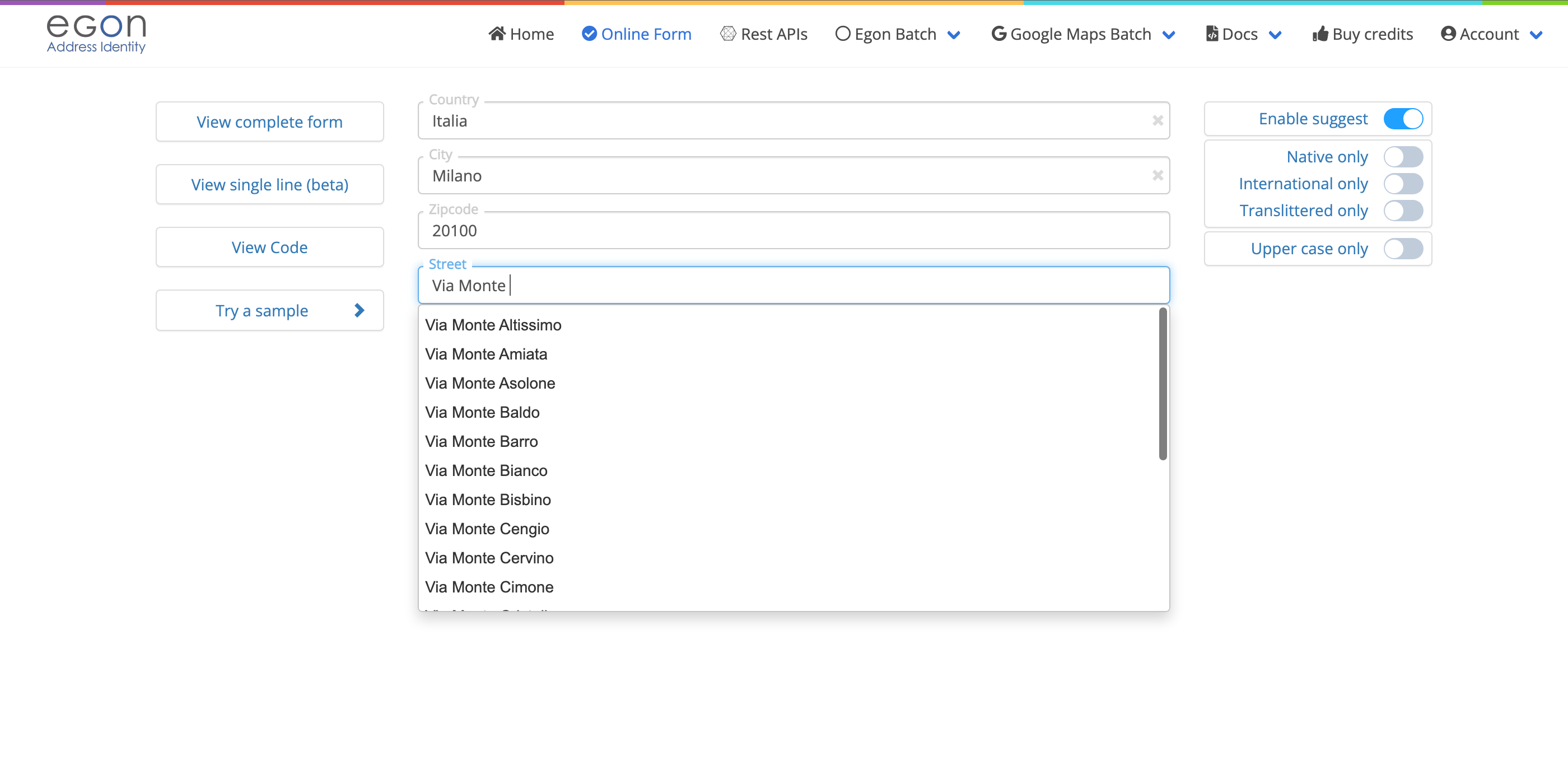
Example request:
curl --request GET \
--get "https://api.egon.com/v3/suggest/street?query=Via+Roma&iso3=ITA&restrict_id=38000004730" \
--header "Authorization: Bearer {YOUR_APP_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$response = $client->get(
'https://api.egon.com/v3/suggest/street',
[
'headers' => [
'Authorization' => 'Bearer {YOUR_APP_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'query'=> 'Via Roma',
'iso3'=> 'ITA',
'restrict_id'=> '38000004730',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://api.egon.com/v3/suggest/street"
);
const params = {
"query": "Via Roma",
"iso3": "ITA",
"restrict_id": "38000004730",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"Authorization": "Bearer {YOUR_APP_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
[
{
"level": "street",
"id": 38000073652,
"region": "Veneto",
"province": "Verona",
"province_code": "VR",
"city": "Verona",
"street": "Via Roma",
"zipcode": "37121"
},
{
"level": "street",
"id": 38001142331,
"region": "Veneto",
"province": "Verona",
"province_code": "VR",
"city": "Verona",
"street": "Via Romagna",
"zipcode": "37134"
},
{
"level": "street",
"id": 38000073653,
"region": "Veneto",
"province": "Verona",
"province_code": "VR",
"city": "Verona",
"street": "Via Beniamino Romagnoli",
"zipcode": "37139"
}
]
Example response (401, Unauthorized):
{
"error": {
"code": 401,
"message": "You did not provide an API key. You need to provide your API key in the Authorization header, using Bearer auth (e.g. 'Authorization: Bearer {token}'). See https://account.egon.com/docs for details."
}
}
Example response (400, Bad Request):
{
"error": {
"code": 400,
"message": "Missing the 'iso3'."
}
}
Received response:
Request failed with error:
Response
Response Fields
level
string
Territorial level of the element.
id
integer
Element ID.
state
string
(optional) State.
state_code
string
(optional) State code.
region
string
(optional) Region.
region_code
string
(optional) Region code.
province
string
(optional) Province.
province_code
string
(optional) Province code.
city
string
City.
district1
string
(optional) Sub-locality District level 1.
zipcode
string
(optional) Postal code.
street
string
Street name.
Zipcode
requires authentication
Suggestion for zipcode
Example request:
curl --request GET \
--get "https://api.egon.com/v3/suggest/zipcode?query=42023&iso3=ITA&zip_level=L" \
--header "Authorization: Bearer {YOUR_APP_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$response = $client->get(
'https://api.egon.com/v3/suggest/zipcode',
[
'headers' => [
'Authorization' => 'Bearer {YOUR_APP_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'query'=> '42023',
'iso3'=> 'ITA',
'zip_level'=> 'L',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://api.egon.com/v3/suggest/zipcode"
);
const params = {
"query": "42023",
"iso3": "ITA",
"zip_level": "L",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"Authorization": "Bearer {YOUR_APP_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
[
{
"country": "Italia",
"region": "Emilia-Romagna",
"province": "Reggio Emilia",
"level": "city",
"id": 38000005186,
"city": "Cadelbosco di Sopra",
"city_id": 38000005186,
"zipcode": "42023"
},
{
"country": "Italia",
"region": "Emilia-Romagna",
"province": "Reggio Emilia",
"level": "district1",
"id": 38000013982,
"city": "Cadelbosco di Sopra",
"city_id": 38000005186,
"district1": "Argine",
"zipcode": "42023"
},
{
"country": "Italia",
"region": "Emilia-Romagna",
"province": "Reggio Emilia",
"level": "district1",
"id": 38000013983,
"city": "Cadelbosco di Sopra",
"city_id": 38000005186,
"district1": "Seta",
"zipcode": "42023"
},
{
"country": "Italia",
"region": "Emilia-Romagna",
"province": "Reggio Emilia",
"level": "district1",
"id": 38000018193,
"city": "Cadelbosco di Sopra",
"city_id": 38000005186,
"district1": "Villa Seta",
"zipcode": "42023"
},
{
"country": "Italia",
"region": "Emilia-Romagna",
"province": "Reggio Emilia",
"level": "district1",
"id": 38000022111,
"city": "Cadelbosco di Sopra",
"city_id": 38000005186,
"district1": "Zurco",
"zipcode": "42023"
},
{
"country": "Italia",
"region": "Emilia-Romagna",
"province": "Reggio Emilia",
"level": "district1",
"id": 38000027973,
"city": "Cadelbosco di Sopra",
"city_id": 38000005186,
"district1": "Cadelbosco di Sotto",
"zipcode": "42023"
},
{
"country": "Italia",
"region": "Emilia-Romagna",
"province": "Reggio Emilia",
"level": "district1",
"id": 38000030577,
"city": "Cadelbosco di Sopra",
"city_id": 38000005186,
"district1": "Santa Vittoria",
"zipcode": "42023"
},
{
"country": "Italia",
"region": "Emilia-Romagna",
"province": "Reggio Emilia",
"level": "district1",
"id": 38001352004,
"city": "Cadelbosco di Sopra",
"city_id": 38000005186,
"district1": "Argine Vecchio",
"zipcode": "42023"
},
{
"country": "Italia",
"region": "Emilia-Romagna",
"province": "Reggio Emilia",
"level": "district1",
"id": 38001352005,
"city": "Cadelbosco di Sopra",
"city_id": 38000005186,
"district1": "Basetti",
"zipcode": "42023"
},
{
"country": "Italia",
"region": "Emilia-Romagna",
"province": "Reggio Emilia",
"level": "district1",
"id": 38001352006,
"city": "Cadelbosco di Sopra",
"city_id": 38000005186,
"district1": "Cantone",
"zipcode": "42023"
},
{
"country": "Italia",
"region": "Emilia-Romagna",
"province": "Reggio Emilia",
"level": "district1",
"id": 38001352007,
"city": "Cadelbosco di Sopra",
"city_id": 38000005186,
"district1": "La Madonnina",
"zipcode": "42023"
},
{
"country": "Italia",
"region": "Emilia-Romagna",
"province": "Reggio Emilia",
"level": "district1",
"id": 38001352008,
"city": "Cadelbosco di Sopra",
"city_id": 38000005186,
"district1": "Ponte Forca",
"zipcode": "42023"
},
{
"country": "Italia",
"region": "Emilia-Romagna",
"province": "Reggio Emilia",
"level": "district1",
"id": 38001352009,
"city": "Cadelbosco di Sopra",
"city_id": 38000005186,
"district1": "Quarti",
"zipcode": "42023"
},
{
"country": "Italia",
"region": "Emilia-Romagna",
"province": "Reggio Emilia",
"level": "district1",
"id": 38001352010,
"city": "Cadelbosco di Sopra",
"city_id": 38000005186,
"district1": "Stazione Bosco Sotto",
"zipcode": "42023"
},
{
"country": "Italia",
"region": "Emilia-Romagna",
"province": "Reggio Emilia",
"level": "district1",
"id": 38001352011,
"city": "Cadelbosco di Sopra",
"city_id": 38000005186,
"district1": "Villa Argine",
"zipcode": "42023"
},
{
"country": "Italia",
"region": "Emilia-Romagna",
"province": "Reggio Emilia",
"level": "district1",
"id": 38001470449,
"city": "Cadelbosco di Sopra",
"city_id": 38000005186,
"district1": "Corte Cantina",
"zipcode": "42023"
},
{
"country": "Italia",
"region": "Emilia-Romagna",
"province": "Reggio Emilia",
"level": "district1",
"id": 38001470450,
"city": "Cadelbosco di Sopra",
"city_id": 38000005186,
"district1": "La Tomba",
"zipcode": "42023"
},
{
"country": "Italia",
"region": "Emilia-Romagna",
"province": "Reggio Emilia",
"level": "district1",
"id": 38001470451,
"city": "Cadelbosco di Sopra",
"city_id": 38000005186,
"district1": "Florida",
"zipcode": "42023"
},
{
"country": "Italia",
"region": "Emilia-Romagna",
"province": "Reggio Emilia",
"level": "district1",
"id": 38001470452,
"city": "Cadelbosco di Sopra",
"city_id": 38000005186,
"district1": "Molino del Traghettino",
"zipcode": "42023"
},
{
"country": "Italia",
"region": "Emilia-Romagna",
"province": "Reggio Emilia",
"level": "district1",
"id": 38001470453,
"city": "Cadelbosco di Sopra",
"city_id": 38000005186,
"district1": "Traghettino",
"zipcode": "42023"
}
]
Example response (401, Unauthorized):
{
"error": {
"code": 401,
"message": "You did not provide an API key. You need to provide your API key in the Authorization header, using Bearer auth (e.g. 'Authorization: Bearer {token}'). See https://account.egon.com/docs for details."
}
}
Received response:
Request failed with error:
Response
Response Fields
level
string
Territorial level of the element.
id
integer
Element ID.
country
string
Country name.
state
string
(optional) State.
state_code
string
(optional) State code.
region
string
(optional) Region.
region_code
string
(optional) Region code.
province
string
(optional) Province.
province_code
string
(optional) Province code.
city
string
City.
city_id
integer
(optional) City ID of the element.
district1
string
(optional) Sub-locality District level 1.
street
string
(optional) Street name.
number
string
(optional) House number.
exponent
string
(optional) Exponent.
zipcode
string
Postal code.
building
string
(optional) Building name (only for GBR and IRL).
subbilding
string
(optional) Sub-Building information (only for GBR and IRL).
organization
string
(optional) Organization name (only for GBR and IRL).
Validation V3 - OLD
Validation API V3
requires authentication
This API will be deprecated starting 1 June 2024. Check the new Validation V4!
Example request:
curl --request GET \
--get "https://api.egon.com/v3/validation?country_code=ITA&city=verona&addr1=via+antonio+pacinotti+204" \
--header "Authorization: Bearer {YOUR_APP_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$response = $client->get(
'https://api.egon.com/v3/validation',
[
'headers' => [
'Authorization' => 'Bearer {YOUR_APP_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'country_code'=> 'ITA',
'city'=> 'verona',
'addr1'=> 'via antonio pacinotti 204',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://api.egon.com/v3/validation"
);
const params = {
"country_code": "ITA",
"city": "verona",
"addr1": "via antonio pacinotti 204",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"Authorization": "Bearer {YOUR_APP_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
[
{
"result": {
"result_fl": "1"
},
"output_singleLine": "Via Antonio Pacinotti,204, Verona, VR, Veneto, 37135, Italia",
"output_smart": {
"country": "Italia",
"adm_level_main": "Verona",
"city": "Verona",
"addr": "Via Antonio Pacinotti,204",
"zipcode": "37135"
},
"output_complete": {
"country": "Italia",
"state": "",
"region": "Veneto",
"province": "Verona",
"city": "Verona",
"sub_locality_main": "",
"sub_locality1": "",
"sub_locality2": "",
"sub_locality3": "",
"postal_town": "VERONA",
"zipcode": "37135",
"addr1": "Via Antonio Pacinotti,204",
"addr2": "",
"street_name": "Via Antonio Pacinotti",
"house_number": "204",
"house_number_ext": "",
"building": "",
"subbuilding": "",
"organization": "",
"org_department": "",
"near_by": "",
"care_of": "",
"po_box": "",
"out_lng": "380"
},
"egon_codes": {
"egon_city_code": {
"lValue": 38000004730
},
"egon_sbl_code": {
"lValue": 0
},
"egon_str_code": {
"lValue": 38000073526
},
"egon_key_code": {
"lValue": 0
}
},
"adm_codes": {
"nr9Ele": {
"lValue": 12
},
"areaCode": [
{
"code_type": "50137",
"code_value": "VR"
},
{
"code_type": "30000",
"code_value": "L781"
},
{
"code_type": "30021",
"code_value": "023091"
},
{
"code_type": "30022",
"code_value": "005"
},
{
"code_type": "30030",
"code_value": "11700"
},
{
"code_type": "30050",
"code_value": "0777000"
},
{
"code_type": "30060",
"code_value": "086"
},
{
"code_type": "30061",
"code_value": "660999"
},
{
"code_type": "30070",
"code_value": "ITA"
},
{
"code_type": "30071",
"code_value": "IT"
},
{
"code_type": "30072",
"code_value": "380"
},
{
"code_type": "30114",
"code_value": "ITALIA"
}
]
},
"geocoding": {
"geo_level": "A41-261",
"long_lat": "45.40785120 , 10.96922180"
}
},
{
"WP9STC": {
"lValue": 0
}
}
]
Example response (401, Unauthorized):
{
"error": {
"code": 401,
"message": "You did not provide an API key. You need to provide your API key in the Authorization header, using Bearer auth (e.g. 'Authorization: Bearer {token}'). See https://account.egon.com/docs for details."
}
}
Received response:
Request failed with error:
Response
Response Fields
result.result_fl
string
Address Validation result.
- 0 = Address validated - All levels
- 1 = Address validated - Street level
- 2 = Address validated - City/Locality level
- 3 = Address not validated
output_singleLine
string
Complete address on single line. It contains all territorial levels including the country name.
output_smart.country
string
Country name.
output_smart.administrative_level
string
Main administrative level above the City.
output_smart.city
string
City.
output_smart.addr
string
Address.
output_smart.zipcode
string
Postal code.
output_complete.country
string
Country.
output_complete.state
string
State. Not every Country exhibits this territorial level.
output_complete.region
string
Region. Not every Country exhibits this territorial level.
output_complete.province
string
Province. Not every Country exhibits this territorial level.
output_complete.city
string
City.
output_complete.sub_locality_main
string
Sub-locality District main level. It contains the main sub-locality among the 3 sub_locality levels.
output_complete.locality
string
Sub-locality District level 1. Not every Country exhibits this territorial level.
output_complete.locality2
string
Sub-locality District level 2. Not every Country exhibits this territorial level.
output_complete.locality3
string
Sub-locality District level 3. Not every Country exhibits this territorial level.
output_complete.postal_town
string
Postal Town. Not every Country exhibits postal towns.
output_complete.zipcode
string
Postal code.
output_complete.addr1
string
Complete address. It contains the house number and any additional address information.
output_complete.addr2
string
Secondary address. Only for UK
output_complete.street_name
string
Street name.
output_complete.hn
string
House number + Exponent.
output_complete.hn_ext
string
House number extra information.
output_complete.building
string
Building name. Only for UK.
output_complete.subbuilding
string
Sub-Building name. Only for UK.
output_complete.organization
string
Organization Name. Only for UK.
output_complete.org_department
string
Organization Department. Only for UK.
output_complete.near_by
string
Near by.
output_complete.care_of
string
Care of.
output_complete.po_box
string
PO Box.
output_complete.out_lng
string
Output language. It indicates the language code (ISO 3166-1 numeric for native language) or the transliteration code.
egon_codes.egon_key_code
integer
Egon key code.
egon_codes.egon_str_code
integer
Egon street code.
egon_codes.egon_city_code
integer
Egon city code.
egon_codes.egon_sbl_code
integer
Egon subocality code.
adm_codes.code_type
string
Code type. It may contain statistical/administrative types of code.
adm_codes.code_value
string
Code value. Statistical/administrative code value.
geocoding.geo_level
string
Geocoding quality indicator.
geocoding.long_lat
string
Longitude and latitude. Expressed in degrees on WGS84 coordinates system format: +YY.YYYYYYY , +XX.XXXXXXX
Validation V4
Validation API V4 Address
requires authentication
Example request:
curl --request POST \
"https://api.egon.com/v4/validation/address" \
--header "Authorization: Bearer {YOUR_APP_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json" \
--data "{
\"par\": {
\"iso3\": \"ITA\",
\"geo\": \"S\"
},
\"data\": {
\"address\": {
\"city\": \"Verona\",
\"street\": \"Via Pacinotti 4b\"
}
}
}"
$client = new \GuzzleHttp\Client();
$response = $client->post(
'https://api.egon.com/v4/validation/address',
[
'headers' => [
'Authorization' => 'Bearer {YOUR_APP_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'json' => [
'par' => [
'iso3' => 'ITA',
'geo' => 'S',
],
'data' => [
'address' => [
'city' => 'Verona',
'street' => 'Via Pacinotti 4b',
],
],
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://api.egon.com/v4/validation/address"
);
const headers = {
"Authorization": "Bearer {YOUR_APP_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
let body = {
"par": {
"iso3": "ITA",
"geo": "S"
},
"data": {
"address": {
"city": "Verona",
"street": "Via Pacinotti 4b"
}
}
};
fetch(url, {
method: "POST",
headers,
body: JSON.stringify(body),
}).then(response => response.json());
Example response (200):
{
"data": {
"address": {
"standard": {
"iso3": "ITA",
"country": "Italia",
"region": "Veneto",
"province": "Verona",
"province_code": "VR",
"city": "Verona",
"zipcode": "37135",
"street_type": "Via",
"street_name": "Antonio Pacinotti",
"street": "Via Antonio Pacinotti",
"address": "Via Antonio Pacinotti,4/B",
"full_address": "Via Antonio Pacinotti,4/B, Verona, VR, Veneto, 37135, Italia",
"hn": "4/B",
"postal_town1": "VERONA",
"adm_code": [
{
"iso3": "ITA",
"type": "50137",
"value": "VR"
},
{
"iso3": "ITA",
"type": "30030",
"value": "11700"
},
{
"iso3": "ITA",
"type": "30000",
"value": "L781"
},
{
"iso3": "ITA",
"type": "30021",
"value": "023091"
},
{
"iso3": "ITA",
"type": "30060",
"value": "086"
},
{
"iso3": "ITA",
"type": "30061",
"value": "660999"
},
{
"iso3": "ITA",
"type": "30050",
"value": "0777000"
},
{
"iso3": "ITA",
"type": "30071",
"value": "IT"
},
{
"iso3": "ITA",
"type": "30070",
"value": "ITA"
},
{
"iso3": "ITA",
"type": "30072",
"value": "380"
},
{
"iso3": "ITA",
"type": "30114",
"value": "ITALIA"
},
{
"iso3": "ITA",
"type": "30022",
"value": "005"
}
],
"egon_code": {
"city": 38000004730,
"street": 38000073526,
"hn": 380100008326045
}
},
"smart": {
"country": "Italia",
"administrative_level": "Verona",
"city": "Verona",
"address": "Via Antonio Pacinotti,4/B",
"zipcode": "37135"
}
},
"geo": {
"lat_long": "45.40616800, 10.97379600",
"geo_level": "A41-111",
"census_code": "0230910000922"
},
"postal": {
"row4": "VIA ANTONIO PACINOTTI 4/B",
"row5": "37135 VERONA VR"
}
},
"quality": {
"address": {
"locality": {
"flag": "0",
"code": 0,
"description": "Ok"
},
"street": {
"flag": "1",
"code": 801,
"description": "Candidato strada con parole in meno"
},
"hn": {
"flag": "0",
"code": 0,
"description": "Ok"
}
}
},
"system": {
"ret_code": 0,
"des_ret_code": "Ok"
}
}
Received response:
Request failed with error:
Response
Response Fields
data
object
data.address
object
Validated address data
data.address.standard
object
Standard address format
data.address.standard.iso3
string
Country code (ISO 3166-1 alpha-3)
data.address.standard.country
string
Country description
data.address.standard.state
string
State description
data.address.standard.state_code
string
State code
data.address.standard.region
string
Region description
data.address.standard.region_code
string
Region code
data.address.standard.province
string
Province description
data.address.standard.province_code
string
Province code
data.address.standard.city
string
City description
data.address.standard.locality
string
District description
data.address.standard.locality2
string
District2 description
data.address.standard.locality3
string
District3 description
data.address.standard.zipcode
string
Zipcode
data.address.standard.full_address
string
Full address
data.address.standard.street_type
string
Dug (E.g. Via)
data.address.standard.street_name
string
Street description (E.g. Garibaldi)
data.address.standard.street
string
Dug + street description (E.g. Via Pacinotti 4b)
data.address.standard.address
string
Dug + street description + number (E.g. Via Pacinotti 4b)
data.address.standard.street_type_2
string
Dug 2 (E.g. Via)
data.address.standard.street_name_2
string
Street 2 description (E.g. Garibaldi)
data.address.standard.street_2
string
Dug 2 + Street 2 description (E.g. Via Pacinotti 4b)
data.address.standard.hn
string
House number
data.address.standard.hn_ext
string
House number other information
data.address.standard.hn_2
string
House number 2
data.address.standard.building
string
Building
data.address.standard.subbuilding
string
Subbuilding
data.address.standard.postal_town1
string
Postal area – city
data.address.standard.postal_town2
string
Postal area - locality
data.address.standard.adm_code
string[]
Administrative codes
data.address.standard.adm_code[].iso3
string
Country ISO code (ITA for Italy)
data.address.standard.adm_code[].type
string
Type of code
data.address.standard.adm_code[].value
string
Code description
data.address.standard.cnd
string[]
Candidates
data.address.standard.cnd[].province
string
Candidate: Province
data.address.standard.cnd[].city
string
Candidate: City
data.address.standard.cnd[].locality
string
Candidate: City/Town
data.address.standard.cnd[].street
string
Candidate: Street
data.address.standard.cnd[].zipcode
string
Candidate: Zipcode
data.address.standard.cnd[].street_code
integer
Candidate: Street code
data.address.standard.egon_code
object
Egon codes
data.address.standard.egon_code.city
integer
City code
data.address.standard.egon_code.street
integer
Street code
data.address.standard.egon_code.hn
integer
House number code
data.address.smart
object
Smart address format
data.address.smart.country
string
Country
data.address.smart.administrative_level
string
Administrative level
data.address.smart.city
string
City
data.address.smart.street
string
Street
data.address.smart.zipcode
string
Zipcode
data.geo
object
Geocoding
data.geo.lat_long
string
Latitude, longitude
data.geo.geo_level
string
Geocoding quality indicator - Geocoding level
data.geo.census_code
string
Micro-zone
data.geo.census_lat_long
string
Micro-zone latitude, longitude
data.postal
object
Postal information
data.postal.row1
string
Postal row 1
data.postal.row2
string
Postal row 2
data.postal.row3
string
Postal row 3
data.postal.row4
string
Postal row 4
data.postal.row5
string
Postal row 5
data.postal.row6
string
Postal row 6
data.postal.row7
string
Postal row 7
quality
object
Quality indicators
quality.address
object
Address quality indicators
quality.address.locality
object
Locality quality indicators
quality.address.locality.flag
string
Address validation result flag (Locality)
It can have the following values:
- 0 Validated without changes.
- 1 Informational: Validated with changes
- 2/3 Not validated
quality.address.locality.code
integer
Warning/Error code (Locality)
quality.address.locality.description
string
Description (Locality)
Locality response codes:
- 201 - No data - locality
- 202 - Locality not found
- 203 - Ambiguous locality
- 212 - Locality not found no candidates
- 701 - Locality candidate found
quality.address.street
object
Street quality indicators
quality.address.street.flag
string
Address validation result flag (Street)
It can have the following values:
- 0 Validated without changes.
- 1 Informational: Validated with changes
- 2/3 Not validated
quality.address.street.code
integer
Warning/Error code (Street)
quality.address.street.description
string
Description
Street response codes:
- 301 - No data - street
- 302 - Street not found
- 303 - Ambiguous street
- 305 - Postcode not attributable for the house number
- 312 - Street candidates not found
- 801 - Street candidate found
quality.address.hn
object
House number quality indicators
quality.address.hn.flag
string
Address validation result flag (House number)
It can have the following values:
- 0 Validated without changes.
- 1 Informational: Validated with changes
- 2/3 Not validated
quality.address.hn.code
integer
Warning/Error code (House number)
quality.address.hn.description
string
Description
House number response codes:
- 590 - Certification not active
system
object
System error
system.ret_code
integer
System code:
- 0 = no blocking error
- <> 0 blocking error code
system.des_ret_code
string
Error description
Validation API V4 Emails
requires authentication
Email normalization. Maximum of 5 emails in input.
Example request:
curl --request POST \
"https://api.egon.com/v4/validation/emails" \
--header "Authorization: Bearer {YOUR_APP_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json" \
--data "{
\"par\": {
\"iso3\": \"ITA\"
},
\"data\": {
\"emails\": [
{
\"email\": \"mario.rossi@gmail.com\"
}
]
}
}"
$client = new \GuzzleHttp\Client();
$response = $client->post(
'https://api.egon.com/v4/validation/emails',
[
'headers' => [
'Authorization' => 'Bearer {YOUR_APP_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'json' => \Symfony\Component\VarExporter\Internal\Hydrator::hydrate(
$o = [
clone (\Symfony\Component\VarExporter\Internal\Registry::$prototypes['stdClass'] ?? \Symfony\Component\VarExporter\Internal\Registry::p('stdClass')),
],
null,
[
'stdClass' => [
'email' => [
'mario.rossi@gmail.com',
],
],
],
[
'par' => [
'iso3' => 'ITA',
],
'data' => [
'emails' => [
$o[0],
],
],
],
[]
),
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://api.egon.com/v4/validation/emails"
);
const headers = {
"Authorization": "Bearer {YOUR_APP_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
let body = {
"par": {
"iso3": "ITA"
},
"data": {
"emails": [
{
"email": "mario.rossi@gmail.com"
}
]
}
};
fetch(url, {
method: "POST",
headers,
body: JSON.stringify(body),
}).then(response => response.json());
Example response (200):
{
"data": {
"emails": [
{
"email": "mario.rossi@gmail.com"
}
]
},
"quality": {
"emails": [
{
"email1": {
"flag": "1",
"code": 991
}
}
]
},
"system": {
"ret_code": 0,
"des_ret_code": "Ok"
}
}
Received response:
Request failed with error:
Response
Response Fields
data.emails[].email
string
quality.emails.email[i].flag
string
i must be between 1 and 5
Signal level. It can have the following values:
- 0 Validated without changes.
- 1 Informational: Validated with changes
- 2/3 Not validated
quality.emails.email[i].code
integer
Signal code
system.ret_code
integer
System code:
- 0 = no blocking error
- <> 0 blocking error code
system.des_ret_code
string
Error description
Response errors:
OK with corrections/warnings – Flag = 1
- 463 - Double points
- 464 - IP Formatted email
- 465 - Points found
- 466 - Valid Domain
- 467 - Email with generic National first level domain
- 468 - Email with national first level domain
- 469 - Email with official domain
- 980 - First level domain not certified
- 981 - Forced @
- 982 - Substitution @
- 983 - Email modified
- 984 - Domain modified
- 985 - Fake email
- 986 - Email starting with special characters
- 987 - URL in email
- 988 - Too many emails
- 989 - Additional information found
- 991 - Certified domain
Errors – Flag = 2/3
- 460 - Format not valid
- 461 - Email format not recognized
- 462 - Unknown domain
- 470 - Not valid domain
- 401 - Email not present
- 402 - Wrong email format
- 428 - Invalid characters
- 443 - Wrong domain
- 454 - Wrong I° level domain
- 455 - Wrong domain
- 456 - @ not present
- 457 - II° level domain not present
- 458 - Domain not certified